Creating a Flash Kiosk: Team Architecture and Organisation
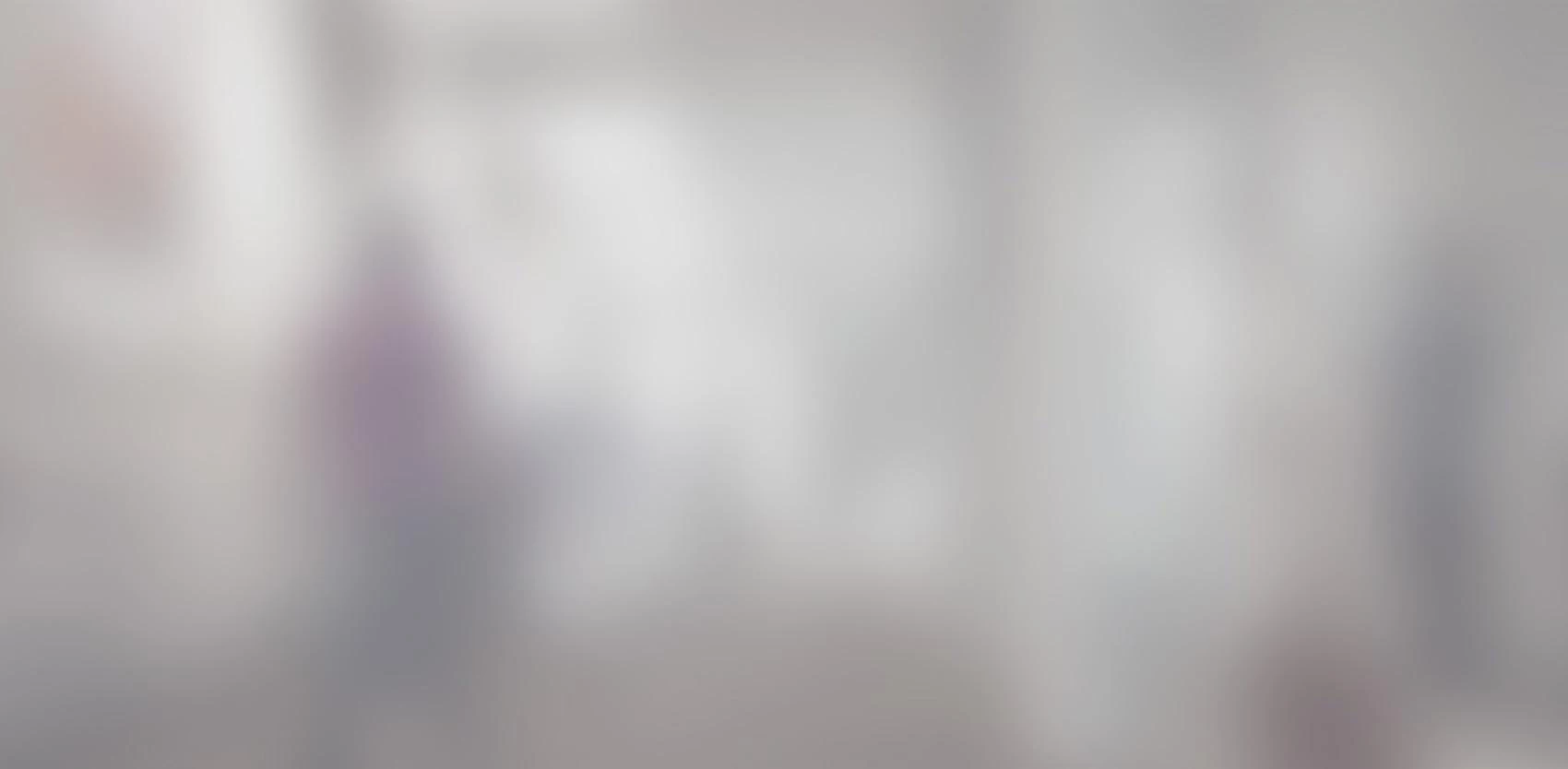
BackgroundThe idea was to create an iPad-controled game on an advertising screen (kiosk). This type of project required the User Interface to be top-notch.Since the game involved animation and graphics, we chose Adobe’s Flash technology.Architecture and TeamThe project is based on three components:Therefore, our production team was made up of:
Background
The idea was to create an iPad-controled game on an advertising screen (kiosk). This type of project required the User Interface to be top-notch.
Since the game involved animation and graphics, we chose Adobe’s Flash technology.
Architecture and Team
The project is based on three components:
Therefore, our production team was made up of:
- 2 User Interface/User Experience (UI/UX) designers,
- 1 iOS developer for the iPad component,
- 1 developer for the Game Engine component.
Interconnected Components = Interactive Team
Since the iPad and the Game Engine had to communicate wirelessly, logic dictated the use of a WiFi connection, turning the Game Engine into a de facto server (TCP Socket).
The “Display” is developed in Flash ActionScript 3. For it to connect to the Game Engine entity, we had several options:
- Develop the Game Engine in ActionScript;
- Communicate using pipes on file handles;
- Develop a second TCP Socket.
We chose the third option, for the following reasons:
- Better separation between entities, for better workload distribution among team members.
- Greater flexibility in the use of Game Engine technology (C#, ASP, Python, PHP, NodeJS, …), and therefore in the type of developer needed.
- Interface homogeneity.
- Standard multiplatform link (some of our team members worked with OS X, others with Windows 7).
- Flexibility in the physical location and number of entities (ability to deal with more than one iPad concurrently, for example).
Here is the architecture design:
Creating a TCP client in Flash AS3
Here is an example of a TCP client:
import flash.net.Socket;
public class GameServer {
private var mSocket: Socket = null;
private function closeHandler(event: Event): void {
trace("connection closed");
}
private function connectHandler(event: Event): void {
trace("connected");
}
private function socketDataHandler(event: ProgressEvent): void {
trace("data rceived");
}
public function GameServer(listener:IGameServerServices)
{
mSocket = new Socket();
mSocket.addEventListener(Event.CLOSE, closeHandler);
mSocket.addEventListener(Event.CONNECT, connectHandler);
mSocket.addEventListener(ProgressEvent.SOCKET_DATA, socketDataHandler);
mSocket.connect(CONFIG::SERVER, CONFIG::PORT);
}
}
Notice that Flash natively decodes the JSON format:
var json = JSON.parse("{\"test\"":true}"");
Looks simple at first glance, but take a second look: the server must answer a Flash request to authorize the connnection.
So, when Flash sends a “
$contents = "\n";
$contents .= " \n";
$contents .= " \n\0";
$client->send($contents);
Don’t forget the “\0” at the end!
The Game Engine
As explained above, we had a wide choice of technologies. To keep things simple, we chose PHP.
Here is the “composer.json” description of the packages used:
{
"autoload": {
"psr-0": {
"MyApp": "src"
}
},
"require": {
"cboden/ratchet": "0.3.*"
}
}
Notice that the PHP Game Engine is also responsible for responding to Flash (HTTP/GET) requests to load dynamic images.
Flash, an elegant solution to interact with the UI/UX team
An interesting lesson learned on this project was the way in which the UI/UX team was an integral part of the development phase.
Often, a UI/UX team studies a project, then supplies the assets used by the integrator, who bridges software development and graphics. This is constraining in the following ways:
- The integrator has to reproduce the designers’ work as well as possible, depending on the technology used.
- Since there is a difference between what the UI/UX team designs and what is actually integratable, the final result is not quite what it should be.
- Intermediaries limit creativity.
But that wasn’t a problem in this case! The UI team produced the actual Flash project directly, and their interaction with code was limited to a couple of “movieClip.play()” and “movieClip.stop()” lines here and there.
The UI integration phase was reduced to a bare minimum, and it was great to see the interface constantly improving with every stroke of inspiration of the designers, long after the developers had completely finished coding.